C Program to Reverse a Linked List
#include <stdio.h>
#include <stdlib.h>
struct node {
int data;
struct node *next;
};
struct node *head = NULL;
struct node *createNode() {
struct node *newNode = (struct node *)malloc(sizeof(struct node));
return newNode;
}
void insertNode() {
struct node *temp, *ptr;
temp = createNode();
printf("Enter the data you want to insert: ");
scanf("%d", &temp->data);
temp->next = NULL;
if (head == NULL)
head = temp;
else {
ptr = head;
while (ptr->next != NULL) {
ptr = ptr->next;
}
ptr->next = temp;
}
}
void listReverse() {
struct node *prev = NULL, *current, *nextNode;
current = head;
while (current != NULL) {
nextNode = current->next;
current->next = prev;
prev = current;
current = nextNode;
}
head = prev;
printf("List reversed successfully\n");
}
void viewList() {
struct node *temp = head;
if (temp == NULL) {
printf("List is empty\n");
} else {
printf("List items are: \n");
while (temp != NULL) {
printf("%d\t", temp->data);
temp = temp->next;
}
printf("\n");
}
}
int menu() {
int choice;
printf("\n 1. Add value to the list");
printf("\n 2. Reverse the list");
printf("\n 3. View the list");
printf("\n 4. Exit");
printf("\n Please enter your choice: ");
scanf("%d", &choice);
return choice;
}
int main() {
while (1) {
switch (menu()) {
case 1:
insertNode();
break;
case 2:
listReverse();
break;
case 3:
viewList();
break;
case 4:
exit(0);
default:
printf("Invalid choice\n");
}
}
return 0;
}
Output:
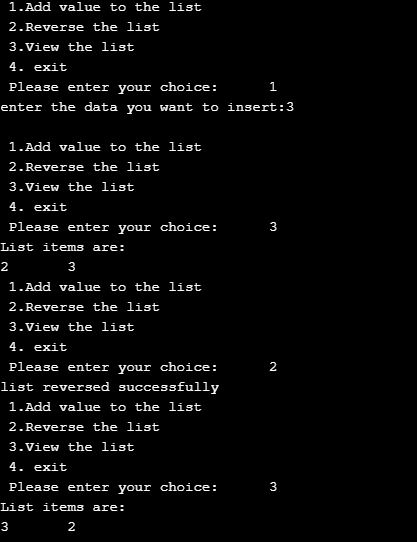
[wpusb]