Deleting a node in linked list From Beginning
#include<conio.h>
#include<stdio.h>
#include<stdlib.h>
struct node{
int data;
struct node *next;
};
struct node *head=NULL;
struct node* createNode(){
struct node *newNode = (struct node *)malloc(sizeof(struct node));
return (newNode);
}
void insertNode(){
struct node *temp,*ptr;
temp=createNode();
printf("enter the data you want to insert:");
scanf("%d",&temp->data);
temp->next=NULL;
if(head==NULL)
head=temp;
else{
ptr=head;
while(ptr->next!=NULL){
ptr=ptr->next;
}
ptr->next=temp;
}
}
void deleteNodeAtBegin(){
if(head==NULL){
printf("list is empty");
}
else{
struct node* temp=head;
head=head->next;
printf("Successfully Deleted %d from Beginning",temp->data);
free(temp);
}
}
void viewList(){
struct node* temp=head;
if(temp==NULL){
printf("list is empty");
}
else{
printf("List is: \n");
while(temp->next!=NULL)
{
printf("%d\t",temp->data);
temp=temp->next;
}
printf("%d \t",temp->data);
}
}
int menu(){
int choice;
printf("\n 1.Add value to the list");
printf("\n 2.Delete node at Begining");
printf("\n 3.Travesre/View List");
printf("\n 4. exit");
printf("\n Please enter your choice: \t");
scanf("%d",&choice);
return(choice);
}
void main(){
while(1){
switch(menu()){
case 1:
insertNode();
break;
case 2:
deleteNodeAtBegin();
break;
case 3:
viewList();
break;
case 4:
exit(0);
default:
printf("invalid choice");
}
getch();
}
}
Output:
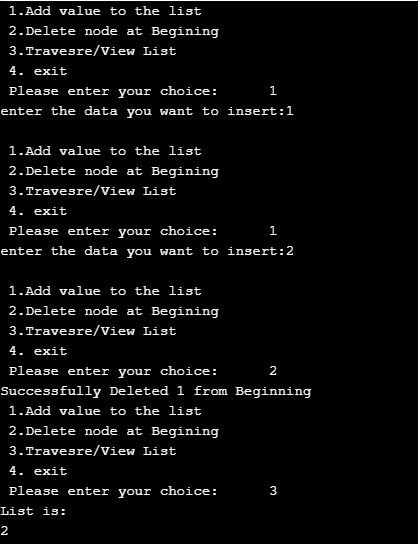
Deleting a node in linked list From End
#include&lt;conio.h&gt; #include&lt;stdio.h&gt; #include&lt;stdlib.h&gt; struct node{ int data; struct node *next; }; struct node *head=NULL; struct node* createNode(){ struct node *newNode = (struct node *)malloc(sizeof(struct node)); return (newNode); } void insertNode(){ struct node *temp,*ptr; temp=createNode(); printf("enter the data you want to insert:"); scanf("%d",&amp;temp-&gt;data); temp-&gt;next=NULL; if(head==NULL) head=temp; else{ ptr=head; while(ptr-&gt;next!=NULL){ ptr=ptr-&gt;next; } ptr-&gt;next=temp; } } void deleteNodeAtEnd(){ struct node *temp,*secondLast; if(head==NULL){ printf("list is empty"); } else{ printf("Successfully Deleted %d from End",temp-&gt;data); temp=head; secondLast=head; while(temp-&gt;next!=NULL){ secondLast=temp; temp=temp-&gt;next; } if(temp==head){ head=NULL; } else{ secondLast-&gt;next=NULL; } free(temp); } } void viewList(){ struct node* temp=head; if(temp==NULL){ printf("list is empty"); } else{ printf("List items are: \n"); while(temp-&gt;next!=NULL) { printf("%d\t",temp-&gt;data); temp=temp-&gt;next; } printf("%d \t",temp-&gt;data); } } int menu(){ int choice; printf("\n 1.Add value to the list"); printf("\n 2.Delete node at End"); printf("\n 3.Travesre/View List"); printf("\n 4. exit"); printf("\n Please enter your choice: \t"); scanf("%d",&amp;choice); return(choice); } void main(){ while(1){ switch(menu()){ case 1: insertNode(); break; case 2: deleteNodeAtEnd(); break; case 3: viewList(); break; case 4: exit(0); default: printf("invalid choice"); } getch(); } }
Output:
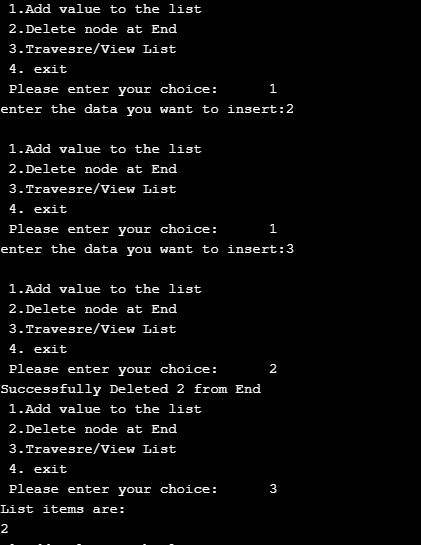
Delete node at given position in a linked list
#include&lt;conio.h&gt; #include&lt;stdio.h&gt; #include&lt;stdlib.h&gt; struct node{ int data; struct node *next; }; struct node *head=NULL; struct node* createNode(){ struct node *newNode = (struct node *)malloc(sizeof(struct node)); return (newNode); } void insertNode(){ struct node *temp,*ptr; temp=createNode(); printf("enter the data you want to insert:"); scanf("%d",&amp;temp-&gt;data); temp-&gt;next=NULL; if(head==NULL) head=temp; else{ ptr=head; while(ptr-&gt;next!=NULL){ ptr=ptr-&gt;next; } ptr-&gt;next=temp; } } void deleteAtLocation(){ struct node *temp1, *temp2; int loc,i; printf("enter the node position you want to delete:"); scanf("%d",&amp;loc); temp1=head; for(i=0;i&lt;loc-1;i++){ temp2=temp1; temp1=temp1-&gt;next; if(temp1==NULL) { printf("\n There are less elements in List"); return; } } temp2-&gt;next = temp1-&gt;next; printf("Successfully Deleted %d from location %d",temp1-&gt;data,loc); free(temp1); printf("\n deleted %d node",loc); } void viewList(){ struct node* temp=head; if(temp==NULL){ printf("list is empty"); } else{ printf("List items are: \n"); while(temp-&gt;next!=NULL) { printf("%d\t",temp-&gt;data); temp=temp-&gt;next; } printf("%d \t",temp-&gt;data); } } int menu(){ int choice; printf("\n 1.Add value to the list"); printf("\n 2.Delete node at given position in a linked list"); printf("\n 3.Travesre/View List"); printf("\n 4. exit"); printf("\n Please enter your choice: \t"); scanf("%d",&amp;choice); return(choice); } void main(){ while(1){ switch(menu()){ case 1: insertNode(); break; case 2: deleteAtLocation(); break; case 3: viewList(); break; case 4: exit(0); default: printf("invalid choice"); } getch(); } }
Output:
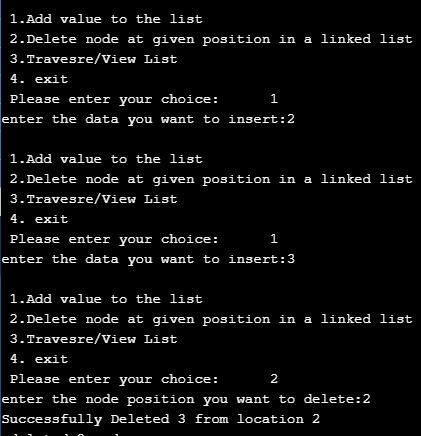