Java Program to implement stack using linked list
public class Main { static class StackNode { int data; StackNode next; StackNode(int data) { this.data = data; } } StackNode root; public void push(int data) { StackNode newNode = new StackNode(data); if (root == null) { root = newNode; } else { StackNode temp = root; root = newNode; newNode.next = temp; } System.out.println("Item pushed into stack = "+data); } public int pop() { int popped; if (root == null) { System.out.println("Stack is Empty"); return 0; } else { popped = root.data; root = root.next; return popped; } } public int peek() { if (root == null) { System.out.println("Stack is empty"); return Integer.MIN_VALUE; } else { return root.data; } } public static void main(String[] args) { Main m = new Main(); m.push(10); m.push(20); m.push(30); System.out.println("Item popped from stack = "+m.pop()); System.out.println(m.peek()+ " Returned by Peek operation"); } }
Output
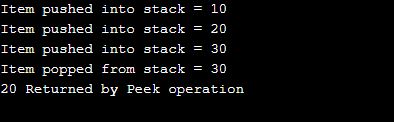